Thermometer using temperature sensor(LM-35)
Generally Thermometer is used to measure the ambient temperature. This article explains you about how to design a simple Thermometer using a temperature sensor.
Theory
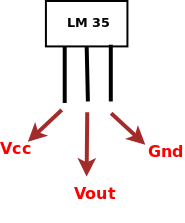
LM-35 is a device which tells you what the ambient temperature is. They use thermistors (temperature sensitive resistors) to measure temperature. If the temperature increases the voltage across the diode increases at the known rate. Technically this is actually the voltage drop between the base and emitter. By precisely amplifying the voltage change, it is easy to generate an analog signal that is directly proportional to temperature.
How to measure Temperature

Temperature is measured by connecting the sensor with an ADC and ZKit-51. The output voltage of the sensor changes with respect to ambient temperature. The output voltage is given to the ADC board and converted into a digital value. The ZKit-51 converts the digital value into precise temperature and displays on the LCD. The output voltage is converted into temperature by using the following formula.
Temperature in degree `C` = `V_o / 10 `
-
`V_o` = Output voltage of the temperature sensor in mV
Features of LM-35
-
Measuring range : -40 to 150 degree C / -40 to 302 degree F.
-
Power supply : 2.7V to 5.5V only, 0.05 mA current draw.
-
Output Voltage : 0.1V (-40 °C) to 2.0V (150 °C) but
-
accuracy decreases after 125 °C
What you need
-
Temperature Sensor(LM-35)
-
ZKit-51 Motherboad
Hardware
Connect the temperature sensor with the ZKit-51 as shown below
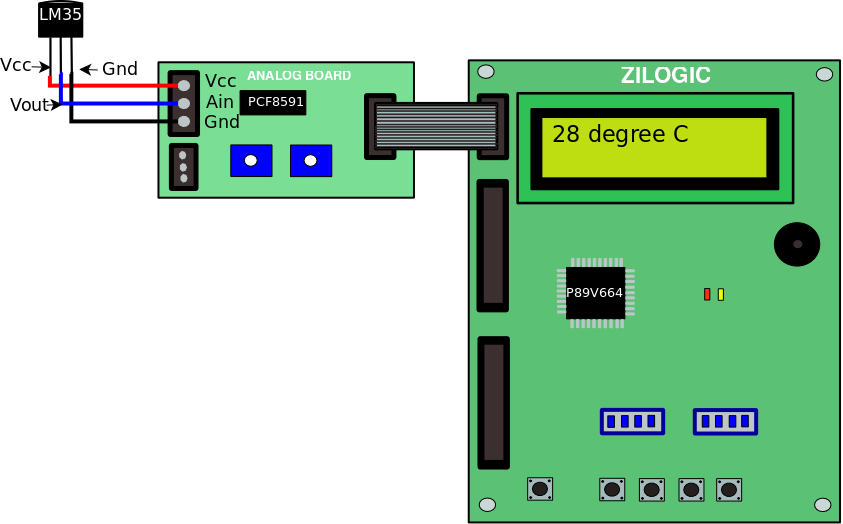
Procedures to connect the sensor with ZKit-51.
-
Connect the Vcc of the sensor with Vcc Phoenix header of the analog board using a wire
-
Connect the ground of the sensor with GND Phoenix header of the analog board using a wire
-
Connect the Vout of the sensor with AIN2 Phoenix header of the analog board using a wire
-
Connect the ZKit-51 Analog Board to the ZKit-51 motherboard SIO header, using a FRC cable.
Software
The sample code for reading the V_out of the sensor and displaying the corresponding temperature is shown below
-
/static/code/zkit-51-thermometer.c[Download the source file]
-
/static/code/zkit-51-thermometer.ihx[Download the hex file for ZKit-51!]
#include <stdio.h>
#include <board.h>
#include <lcd.h>
#include <adc.h>
#include <delay.h>
#define ADC_TO_VOLTAGE 15.803
#define VOL_TO_TEMP 10
#define FIXPT_ARITH 1000
#define PRECISION 500
void putchar(char val)
{
lcd_putc(val);
}
/* Temperature is calculated by reading the value of ADC.
* Output voltage is calculated in mV
*/
unsigned int get_temp(unsigned char val)
{
unsigned int vol;
unsigned int temp;
vol = (val * ADC_TO_VOLTAGE * FIXPT_ARITH + PRECISION) / FIXPT_ARITH;
temp = vol / VOL_TO_TEMP;
return temp;
}
void main()
{
unsigned char adc_val;
unsigned int temp;
board_init();
adc_init();
lcd_init();
delay_init();
adc_enable(2);
while (1) {
lcd_clear();
adc_val = adc_read8(2);
temp = get_temp(adc_val);
printf("%d degree C", temp);
mdelay(300);
}
}